External device properties for HMD¶
The pluginkit can also acquire a set of specified device properties from the HMD device service. The accessed display configuration can be provided to the VR server for the VR app. HMD/Lens driver developer can store their own display configuration in VR DeviceService. Take the above DeviceService sample wvr_simple_deviceservice as an example. The device properties can be accessed in the self-incremental asset folder in SDK/samples/wvr_simple_deviceservice/app/src/main/assets/display. The name of the device properties can be determined by the model number set in the implementation of setupConfig() of the derived HmdDevice instance. The following is a snapshot of a part of a code from MyHmdDevice.java.
package htc.com.vr.sample.simpledevice;
import com.htc.vr.sdk.pluginkit.HmdDevice;
class MyHmdDevice extends HmdDevice {
@Override
public Config setupConfig() {
Config configure = new Config();
configure.trackingSystemName = "HMD Device";
configure.modelNumber = "MDNExmp";
return configure;
}
}
The device properties named with MDNExmp.properties can now be accessed under the assets path. The properties files that have device.properties in the file name are also accessible if the HMD device service doesn’t set the modelNumber.
Required display configuration in HMD device properties
The device properties that have [modelNumber].properties/device.properties in the file name depend on whether the model number is set to the plugin kit. The pluginkit will access the contents of the device properties through:
The display related configurations are listed below.
Required Device Properties | Description
|
Support Version |
---|---|---|
renderconfig.singleBuffer | Enable single buffer rendering or not. Default value
is “true” which is recommended.
ex. renderconfig.singleBuffer=true
|
Since 2.0.23 |
renderconfig.swapInterval | This configuration eventually is passed as the
argument of eglSwapInterval. The synchronous
mode is recommended for Wave runtime by setting
the value “1”.
ex. renderconfig.swapInterval=1
|
Since 2.0.23 |
renderconfig.renderOverfillFactor | This property controls the magnification of FOV,
default value is “1”. Increasing the value expands
mesh size and view frustum. The expansion can fill
the black edge caused by ATW. However, this variation
will result in degrading the image quality.
This is a trade-off.
ex. renderconfig.renderOverfillFactor=1.25
|
Since 2.0.23 |
renderconfig.atwThread | Determine whether creating dedicated thread for ATW.
Default value is “true” which is recommended.
This configuration has been obsolete since SDK 2.1.0
The dedicated thread for ATW is created whether this
configuration is set as true.
ex. renderconfig.atwThread=true
|
Since 2.0.23 until 2.0.37 |
renderconfig.maxMSBeforeVsync | V-Sync timing control parameter for ATW (unit:
milliseconds). Set “0” to delegate Wave runtime
handling the render timing align V-Sync (and half of
V-Sync if single buffer). Set “non-0” will induce
Wave runtime to advance the render timing by the
configured value.
ex. renderconfig.maxMSBeforeVsync=0
|
Since 2.0.23 |
renderconfig.posePredictMS | Pose prediction time for app rendering (unit:
milliseconds). “0” (recommended) means to use the
default prediction time of Wave runtime. “non-0”
means Wave runtime will use this value as prediction
time for app rendering.
ex. renderconfig.posePredictMS=0
|
Since 2.0.23 |
renderconfig.atwPredictMS | Pose prediction time for ATW (unit: milliseconds).
“0” means to use the default prediction time of
Wave runtime. “non-0” means Wave runtime will use this
value as prediction time for ATW rendering.
“0” is recommended.
ex. renderconfig.atwPredictMS=0
|
Since 2.0.23 |
renderconfig.displaySkewCorrectFactor | Display skew correct factor. Value mainly depend on
display scanout latency and scanline type.
For HMD with horizontal display scan from top to bottom
(scanlineType=3), if the user moving head rapidly from
left to right, scene object may look skew in horizontal,
in this case, set displaySkewCorrectFactor to apply to
compensate skew effect in opposite direction. On the
other hand, the user moving head from bow to raise, the
The object may look squash in vertical, in this case,
set displaySquashCorrectFactor to apply to compensate.
displaySkewCorrectFactor and displaySquashCorrectFactor
get exchanging effect if the display scan is vertical.
Zero or negtive means disable.
|
Since 3.1.0 |
renderconfig.displaySquashCorrectFactor | Display squash correct factor. Value mainly depend on
display scanout latency and scanline type.
For HMD with horizontal display scan from top to bottom
(scanlineType=3), if the user moving head rapidly from
left to right, scene object may look skew in horizontal,
in this case, set displaySkewCorrectFactor to apply to
compensate skew effect in opposite direction. On the
other hand, the user moving head from bow to raise, the
The object may look squash in vertical, in this case,
set displaySquashCorrectFactor to apply to compensate.
displaySkewCorrectFactor and displaySquashCorrectFactor
get exchanging effect if the display scan is vertical.
Zero or negtive means disable.
|
Since 3.1.0 |
renderconfig.boundaryBlurRadius | A pair of values for configuring the affected area of
boundary blur. Considered the length of the field of
view as the unit of length, the rendering scene will
start to fade out from the inner radius (the 2nd value)
to outer radius (the 1st value) and everything will be
black besides the outer radius. Both could be given as
any value of floating-point and larger than 1.0 is
accepted. Note: Given renderconfig.boundaryBlurRadius=
0.0, 0.0 will trigger the default effect which is
equivalent to renderconfig.boundaryBlurRadius=1.0, 0.9
|
Since 3.1.0 |
distortionvalue.distanceScaleX | The UV scale in dimension X, keep this value
set as 1 to prevent unexpected visual effect.
ex. distortionvalue.distanceScaleX=1
|
Since 2.0.23 |
distortionvalue.distanceScaleY | The UV scale in dimension Y, set as the value in a ratio
of resolution height to resolution width in one eye
In case that the width and height is 1200x1080, this item
should set as 0.9.
In case that the width and height is 1200x1440, this item
should set as 1.2.
ex. distortionvalue.distanceScaleY=1.2
|
Since 2.0.23 |
distortionvalue.polynomialCoeffsRed | There are eight coefficients in the following formula
for lens distortion. The first of the coefficients is
the offset value usually being set as 0 in WVR platform.
The rest of the coefficients are corresponding
from K0 to K6.
ex. distortionvalue.polynomialCoeffsRed=0,1,0,0,0,2,0,0
|
Since 2.0.23 |
distortionvalue.polynomialCoeffsGreen | There are eight coefficients in the following formula
for lens distortion. The first of the coefficients is
the offset value usually being set as 0 in WVR platform.
The rest of the coefficients are corresponding
from K0 to K6.
ex. distortionvalue.polynomialCoeffsGreen=0,1,0,0,0,2,0,0
|
Since 2.0.23 |
distortionvalue.polynomialCoeffsBlue | There are eight coefficients in the following formula
for lens distortion. The first of the coefficients is
the offset value usually being set as 0 in WVR platform.
The rest of the coefficients are corresponding
from K0 to K6.
ex. distortionvalue.polynomialCoeffsBlue=0,1,0,0,0,2,0,0
|
Since 2.0.23 |
lensvalue.distanceEyeToLens | Distance from eye to the lens (unit: millimeters).
ex. lensvalue.distanceEyeToLens=10
|
Since 2.0.23 |
lensvalue.distanceLensToScreen | Distance from lens to the screen (unit: millimeters).
ex. lensvalue.distanceLensToScreen=30
|
Since 2.0.23 |
lensvalue.lensFocalLength | Focal length of the lens (unit: millimeters).
ex. lensvalue.lensFocalLength=31.123
|
Since 2.0.23 |
lensvalue.ipd | The distance between both center of eyes (unit: meters).
Please refer to the following graph.
ex. lensvalue.ipd=0.0666
|
Since 2.0.23 |
lensvalue.eyeCenterLeft | This is the proportion of the length of the lens where
the center of left eye locates in X and Y direction.
PluginKit currently assumes that the center of eye
locates in the middle of the lens.
Please keep this value set as 0.5, 0.5.
|
Since 2.0.23 |
lensvalue.eyeCenterRight | This is the proportion of the length of the lens where
the center of right eye locates in X and Y direction.
PluginKit currently assumes that the center of eye
locates in the middle of the lens.
Please keep this value set as 0.5, 0.5.
|
Since 2.0.23 |
misc.sensorToHeadFor6Dof | Sensor to center of head offset for 6DoF (unit: meters).
This value depends on the location of sensor module
(or tracking module) on the HMD.
The actual offset should be set in this property since
SDK 2.0.23 until SDK 3.1.6.
ex. misc.sensorToHeadFor6Dof=0,0,0
This configuration has been obsolete since SDK 3.2.0.
Pluginkit takes over the offset calculating.
|
Since 2.0.23 until 3.1.6 |
misc.sensorToHeadFor3Dof | Sensor to center of head offset for 3DoF (unit: meters).
This value depends on the location of sensor module
(or tracking module) on the HMD.
The actual offset should be set in this property since
SDK 2.0.23 until SDK 3.1.6.
ex. misc.sensorToHeadFor3Dof=0,0,0
This configuration has been obsolete since SDK 3.2.0.
Pluginkit takes over the offset calculating.
|
Since 2.0.23 until 3.1.6 |
misc.hasExternalScreen | “false” means that HMD uses primary display;
“true” means that HMD uses external display.
ex. misc.hasExternalScreen=false
|
Since 2.0.23 |
misc.gapBetweenPanels | The distance exists between the dual panels (unit:
millimeters). This gap is horizontally measured across
the ineffective area from the rightmost pixel of the
left panel to the leftmost pixel of the right panel.
Please refer to the following graph.
ex. misc.gapBetweenPanels=6.666
|
Since 2.0.23 |
externalscreen.width | The width of the external screen (unit: pixels). Fill
this property if misc.hasExternalScreen is true.
ex. externalscreen.width=1440
|
Since 2.0.23 |
externalscreen.height | The height of the external screen (unit: pixels).
Fill this property if misc.hasExternalScreen is true.
ex. externalscreen.height=1440
|
Since 2.0.23 |
externalscreen.physicalWidth | The physical width of the external screen, measured in
millimeters. Fill this property if misc.hasExternalScreen
is set as true.
ex. externalscreen.physicalWidth=150
|
Since 2.0.23 |
externalscreen.physicalHeight | The physical height of the external screen, measured in
millimeters. Fill this property if misc.hasExternalScreen
is set as true.
ex. externalscreen.physicalHeight=70
|
Since 2.0.23 |
externalscreen.refreshRate | Frame per second in the external display device.
ex. externalscreen.refreshRate=60
|
Since 2.0.23 |
externalscreen.vsyncTimeOffset | The time delay of vsync is relative to display source.
ex. externalscreen.vsyncTimeOffset=10
|
Since 2.0.23 |
externalscreen.scanlineType | Scanline direction of External display.
1: left to right
2: right to left
3: top to bottom
4: bottom to top
Note: default is 1 (left to right)
|
Since 2.0.23 |
primaryscreen.scanlineType | Scanline direction of primary display.
1: left to right
2: right to left
3: top to bottom
4: bottom to top
Note: default is 1 (left to right)
|
Since 2.0.23 |
Three RGB color components should possess the corresponding lens distortion formula due to distinct wavelength.

The graph below illustrates how to determine the value of lensvalue.ipd and gap distance misc.gapBetweenPanels. In the case of the dual panels, the purple blocks represent the effective areas which are able to show the pixels on the panel. The gray blocks around the rims of the purple blocks represent the ineffective areas. The gap distance should be measured across from one side of the ineffective area to the other.
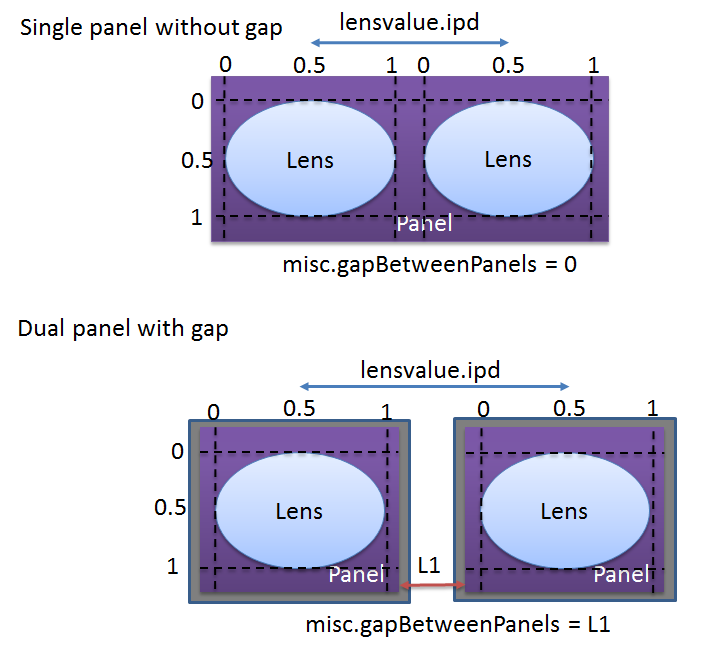
There is another configuration file “device_extras.properties” stored under the same path as “device.properties”. The configurations are optional which means that the default settings are applied if the values are not specified in this file. This configuration file has been supported since version 2.1.8. The initial version of each item is listed as below. The following table elaborates each item.
Extra Device Properties | Description
|
Since version |
---|---|---|
gDefaultRenderTargetWidth | To specify the texture width to customize the render
target size. (unit: pixels). The default is set with
display width in single eye.
ex. gDefaultRenderTargetWidth=1440
|
2.1.8 |
gDefaultRenderTargetHeight | To specify the texture width to customize the render
target size. (unit: pixels). The default is set with
display height.
ex. gDefaultRenderTargetHeight=1440
|
2.1.8 |
gNumberQuadWarpMesh | The number of quadrilateral primitives customizes the
mesh size which affects the quality and performance of
distortion. The default value is 80 in runtime.
ex. gNumberQuadWarpMesh=64
|
2.1.8 |
gForceDisableDistortion | The Boolean value leads to clear all distortion
parameters in runtime which means that the distortion
effect is concealed. The config is set as false in
default to activate the distortion effect.
ex. gForceDisableDistortion=false
|
3.0.2 |
gForceDisableReprojection | The Boolean value leads to reprojection
disabling in ATW. The configuration is set as
false in default to activate the reprojection in ATW.
ex. gForceDisableReprojection=false
|
3.0.2 |
gEnableSetProjectionRaw | This Boolean value activates a series of configurable
setting for frustum. The config is set as false
in default to apply the built-in frustum in runtime.
ex. gEnableSetProjectionRaw=false
|
3.0.2 |
gLeftFrustum_Left gLeftFrustum_Right gLeftFrustum_Top gLeftFrustum_Bottom |
The boundary coordinate of the frustum of the left eye.
Assign these configurations if gEnableSetProjectionRaw
is set as true. Please note that the near plane of the
frustum should not form a shape of square which leads
to incorrect visual effect. Runtime assume to use the
perspective projection matrix to transform these
boundary.
|
3.0.2 |
gRightFrustum_Left gRightFrustum_Right gRightFrustum_Top gRightFrustum_Bottom |
The boundary coordinate of the frustum of the right eye.
Assign these configurations if gEnableSetProjectionRaw
is set as true. Please note that the near plane of the
frustum should not form a shape of square which leads
to incorrect visual effect. Runtime assume to use the
perspective projection matrix to transform these
boundary.
|
3.0.2 |